Lazy load components in Vue3 with Suspense
Suspense is an experimental new feature and the API could change at any time. Learn more about it in theSuspense docs.
Lazy loading is a technique to speed up the performance of a web page. Instead of loading the entire web page and rendering it to the user in one go as in bulk loading, the concept of lazy loading assists in delaying the loading of components until they are needed.
Demo
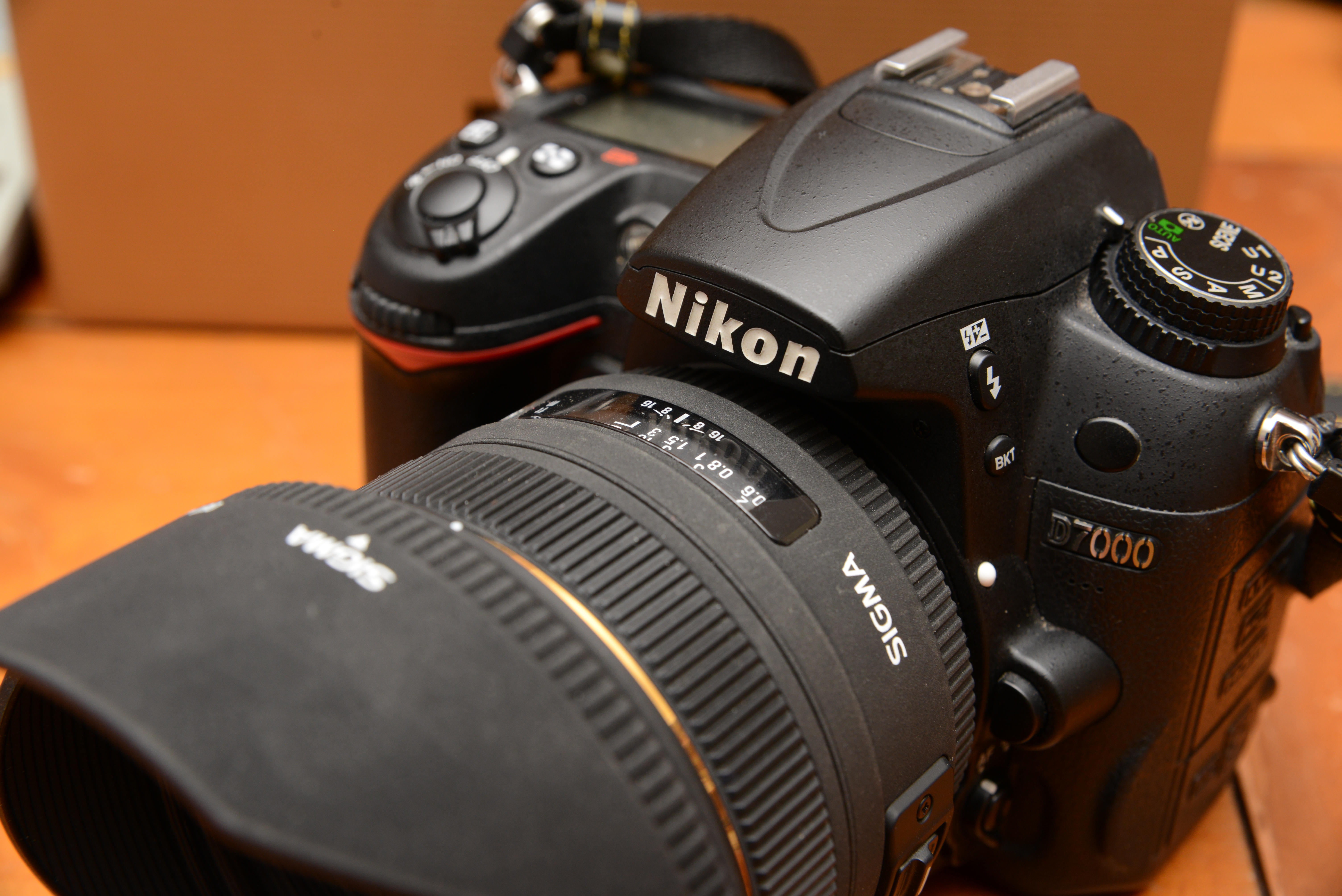
Let’s get to the point. In this example, the file size of the component is huge.
// BigImage.vue
<template>
<img :src="BigImage" class="w-full" alt="big image">
</template>
<script lang="ts" setup>
import BigImage from '~/assets/images/big-image.jpg'
// this will render as async setup()
// faking 4 seconds async job to load the image
const getBigImage = await new Promise(resolve => setTimeout(resolve, 4000))
</script>
Next, in the parent component, we want to lazy load this component. The way we do this is by using thedefineAsyncComponent()
method.
// Parent.vue
<script lang='ts' setup>
const BigImage = defineAsyncComponent (() => import('./BigImage.vue'))
</script>
In the template, we wrap the component with<Suspense>
and pass theasync
component to it. We can omit the#fallback
template if we want to show a loading message.
<template>
<Suspense>
<template #default>
<big-image />
</template>
<template #fallback>
<h1>Lazy Loading...</h1>
</template>
</Suspense>
</template>
Thetemplate #default
is optional. Just a more standard way of doing it.
Look into Devtools under the network tab to see how the component is loaded.
Made with❤by leovoon